- o1.Equals(o2) - equality
- By default, true if o1 and o2 are created by execution of the same new
- Can be redefined in a particular class
- Object.ReferenceEquals(o1, o2) - identity
- True if both o1 and o2 are null, or if they are created by execution of the same new
- Static - cannot be redefined.
- Object.Equals(o1, o2)
- True if Object.ReferenceEquals(o1, o2), or if o1.Equals(o2)
- o1 == o2
- True if both o1 and o2 are null, or if they are created by execution of the same new
- An overloadable operator
ProgMaterials
Friday, August 3, 2018
Equality in C#
Thursday, August 2, 2018
LINQ complexity
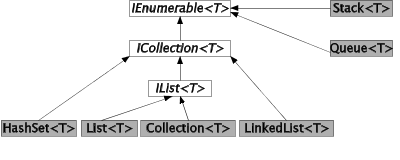
There are very, very few guarantees, but there are a few optimizations:
- Extension methods that use indexed access, such as
ElementAt
,Skip
,Last
orLastOrDefault
, will check to see whether or not the underlying type implementsIList<T>
, so that you get O(1) access instead of O(N). - The
Count
method checks for anICollection
implementation, so that this operation is O(1) instead of O(N). Distinct
,GroupBy
Join
, and I believe also the set-aggregation methods (Union
,Intersect
andExcept
) use hashing, so they should be close to O(N) instead of O(N²).Contains
checks for anICollection
implementation, so it may be O(1) if the underlying collection is also O(1), such as aHashSet<T>
, but this is depends on the actual data structure and is not guaranteed. Hash sets override theContains
method, that's why they are O(1).OrderBy
methods use a stable quicksort, so they're O(N log N) average case.
Tuesday, July 31, 2018
React lifecycle [old]
Constructor:
Bind events
Initialize state.
SetState() won't work here.
ComponentWillMount:
Called before rendering. SetState will not trigger new render.
Git-flow in few words
Постоянные
ветки master и development, остальные временные и должны быть в конечном
итоге удалены.
Feature branches (topic branches):
Порождаются
от development;
Вливаются
в development;
Во время
создания новой ветки может быть не ясно, к какому релизу она будет добавлена.
Covariance and contravariance
Параметры-типы могут быть:
- Инвариантными. Параметр-тип не может изменяться.
- Контравариантными. Параметр-тип может быть
преобразован от класса к
классу, производному от него. В языке C# контравариантный тип
обозначается ключевым словом in. Контравариантный параметр-тип
может появляться только во входной позиции, например, в качестве
аргументов метода. - Ковариантными. Аргумент-тип может быть преобразован от класса к одному из его базовых классов. В языке С# ковариантный тип обозначается ключевым словом out. Ковариантный параметр обобщенного типа может появляться только в выходной позиции, например, в качестве возвращаемого значения метода.
Monday, July 30, 2018
Subscribe to:
Posts (Atom)